In this article, I will show you how to get connected wifi network programmatically with iOS 13.0+.
It is not possible to get all the available wifi networks names and information that are in range of device. But it is only possible to get the SSID
(SSID
is simply the technical term for a network name) of the network that you are currently connected to.
First you need to enable the ‘WiFiAccessApp.entitlements’, Below is the screenshot. this will allow to get connected wifi network.
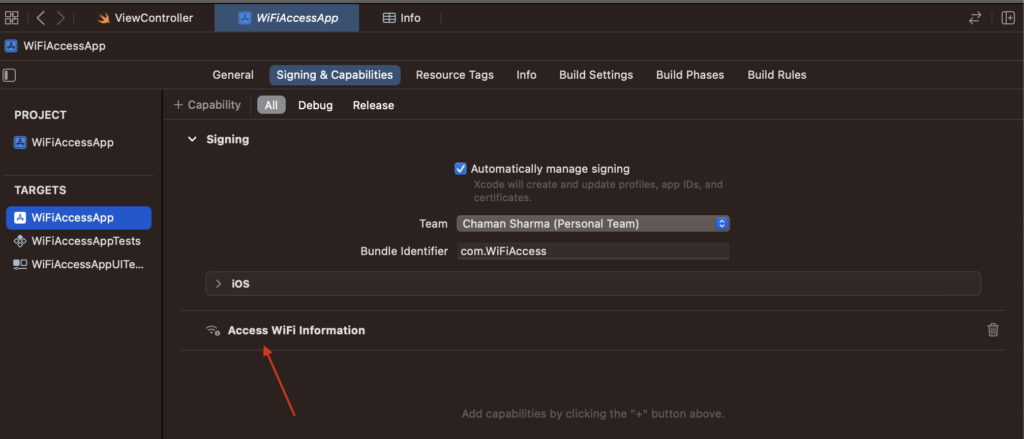
Import below framework in your class –
import SystemConfiguration.CaptiveNetwork
import CoreLocation
you need core location framework to access the location and acces the connected wifi.
Add Location access request in info.plist.
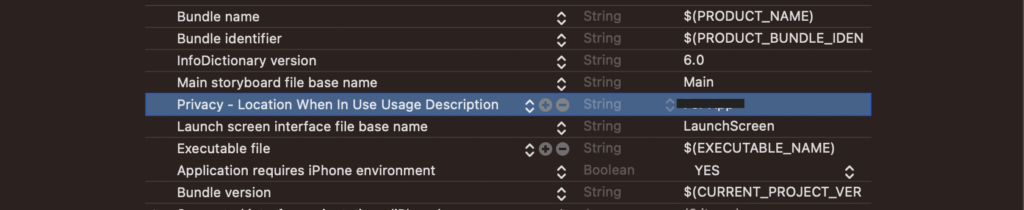
Application is using CoreLocation API and has user’s authorization to access precise location.
Application has used NEHotspotConfiguration API to configure the current Wi-Fi network.
Intialize the LocationManger
var locationManager = CLLocationManager()
override func viewDidLoad() {
super.viewDidLoad()
locationManager.delegate = self
locationManager.requestWhenInUseAuthorization()
locationManager.startUpdatingLocation()
}
Create model class to store network info
struct NetworkInfo {
var interface: String?
var success: Bool?
var bssid: String?
var ssid: String?
}
To get wifi info
func getWifiInfo() {
if let interfaces = CNCopySupportedInterfaces() as NSArray? {
for interface in interfaces {
let interfaceName = interface as! String
let networkInfo = NetworkInfo(interface: interfaceName, success: false, bssid: nil, ssid: nil)
print(networkInfo)
if let interfaceInfo = CNCopyCurrentNetworkInfo(interface as! CFString) as NSDictionary? {
let ssid = interfaceInfo[kCNNetworkInfoKeySSID as String] as? String
let bssid = interfaceInfo[kCNNetworkInfoKeyBSSID as String] as? String
let ssidata = interfaceInfo[kCNNetworkInfoKeySSIDData as String] as? String
}
}
}
}
It is required the after access the location, iOS would give you connected wifi info, Calling ‘getWifiInfo’ after completion acces to location
extension ViewController: CLLocationManagerDelegate {
func locationManager(_ manager: CLLocationManager, didChangeAuthorization status: CLAuthorizationStatus) {
if status == .authorizedWhenInUse {
self.getWifiInfo()
}
}
func locationManager(_ manager: CLLocationManager, didUpdateLocations locations: [CLLocation]) {
self.getWifiInfo()
}
}
In this example:
- The
CNCopySupportedInterfaces()
function is used to obtain a list of supported network interfaces. - The code then iterates through the interfaces and uses
CNCopyCurrentNetworkInfo
to get information about the currently connected Wi-Fi network. - The SSID (Wi-Fi network name) is extracted from the information dictionary.
Keep in mind that this method might return nil
if the device is not connected to a Wi-Fi network or if the information is not available.
Ensure that your app has the necessary permissions to access Wi-Fi information. You may need to add the “Access WiFi Information” capability in your Xcode project settings. Additionally, note that Apple may impose restrictions on accessing Wi-Fi information for certain app types, so check and adhere to Apple’s guidelines and policies.
Reference –
https://developer.apple.com/documentation/networkextension
Hopefully, this post was helpful. Kindly let us know if you need any other help. Thanks